Tutorial: making Gravitech’s MP3 Player add-on work
08 December 2012 at 3:29 pm
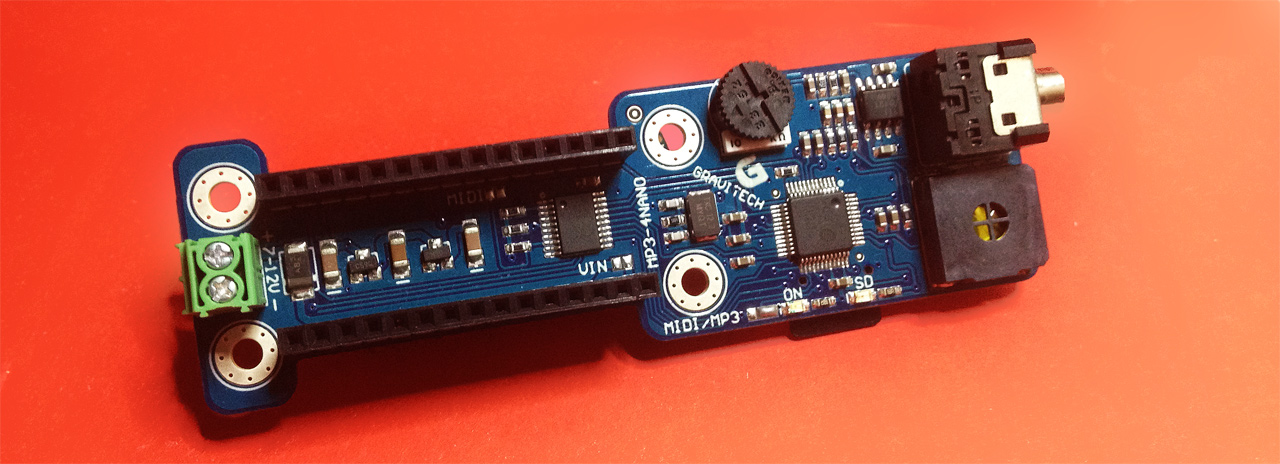
Gravitech is the company that created the Arduino Nano, one of my favorite Arduino’s for prototyping. These are really tiny, but fully breadboard compatible. Just pop it into a breadboard and you’re ready to start making things.
The obvious drawback of this size is that it can’t use standard Arduino Shields, but Gravitech has made their own line of “shields” for the Nano form factor. For a recent project I’m evaluating the MP3-shield and I quickly discovered that Gravitech needs to update the links on the product page as this board can no longer use the code suggested (they’ve done it now).
Making it work
The reason it could use the Sparkfun example, is that it’s using the same decoding chip (VS1053) as Sparkfun’s MP3 Player Shield does. This is a great chip that can not only decode MP3, but also play back Ogg Vorbis, AAC, WMA and MIDI. It can even encode audio if connected correctly.
The problem with the Sparkfun example is that the required SdFat-library has changed. There is no longer a file called “Sd2PinMap.h”, so you can’t do the crucial setting for selecting the SD card. To make the Gravitech MP3 Player add-on for Arduino Nano work, you have to do a few things.
Step 1: Do not download the SdFat-library
The Sparkfun example starts off by saying that you should download and install this from the official URL. Do not do this. We have an easier way.
Step 2: Do not use the Sparkfun example
Instead, use the MUCH better SFEMP3Shield library maintained by Bill Porter (and others). Click the ZIP-download button on the GitHub page (or use GIT if you know how). Unzip the files and place the two folders named “SdFat” and “SFEMP3Shield” in the “libraries” subfolder in your Arduino sketches folder. If this folder is missing, just create it yourself.
The “libraries” folder should be in the following location:
OSX: /Users/username/Documents/Arduino/libraries/
Windows: C:UsersyournameDocumentsArduinolibraries
If you are using the Sparkfun MP3 shield, you can skip the next step. If you are using the Gravitech shield, we need to edit a file for it to work. Do not start your Arduino software yet.
Step 3: Edit SFEMP3ShieldConfig.h
This file is in the “SFEMP3Shield”-folder that you just copied. Open it in a text editor (NOT a word processor!) like TextMate/Textwrangler on Mac or Notepad on Windows. Locate the lines that says “#define GRAVITECH”. This macro was added after I first posted this, so now it’s really easy to set it up. Change this:
#define GRAVITECH 0
into this:
#define GRAVITECH 1
and save the file. That’ll set up the library for the Gravitech MP3 player. What this changes is what the SD_SEL variable (for selecting the SD card) is set to a little further down the same file.
Step 4: Declare the required pins as OUTPUTs
Unless your code explicitly declares the pins of this shield as outputs, you’ll get problems with “floating grounding”. Some pins will flickr between 1 and 0 and this will prevent the SD card from initializing. Sometimes you’ll be able to make it work by just holding the pins, but this’ll be random and not repeatable.
The error you will get is something along the lines of this:
Can't access SD card. Do not reformat. No card, wrong chip select pin, or SPI problem? SD errorCode: 0X1,0X0
To fix it, just add these lines at the top of your code (at the start of void setup() )
pinMode( 2, OUTPUT); pinMode( 3, OUTPUT); pinMode( 4, OUTPUT); pinMode( 6, OUTPUT); pinMode( 7, OUTPUT); pinMode( 8, OUTPUT); pinMode(10, OUTPUT); pinMode(11, OUTPUT); pinMode(12, OUTPUT); pinMode(13, OUTPUT);
Pin 10 isn’t used in the shield, but since the shield is using SPI, it should always be declared as an output.
Step 5: Play audio!
Start the Arduino software and click the Open-button. Locate the SFEMP3Shield-menu and open the example “SMP3Shield_Library_Demo”. Make sure your Board/port settings are correct and compile. Open the Serial Monitor for instructions on how to use the example. When you’ve got the grip on this, study the example to understand how you can implement this into your own app.
Why use SFEMP3Shield rather than the old lib?
As long as you remember to use the old fashioned 8+3 character naming convention (ABCDEFHG.ABC) this library is really good. Many of the Arduino-libraries out there are written by people that don’t understand the general Arduino audience. They are overly complex in both implementation and use. The SFEMP3Shield-library is MUCH better written than the original libraries from Sparkfun. All the complex options are hidden and you’re left with easy to use commands such as setVolume, playTrack, setPlaySpeed and much more. You can also access the ID3 tags of the MP3’s you’re using such as trackTitle, trackArtist and trackAlbum.
If you for some reason run into problems, you can find a good description of Error Codes and other troubleshooting on this page.